- Published on
Quick Git Hooks Setup for Laravel
- Authors
- Name
- Benny Rahmat
- @akunbeben
Author: Benny Rahmat
Language: English
Integrate Git Hooks without headache.
We all know that Git Hooks are specific to each Git repository and are not automatically transferred when cloning a repository. This means that contributors need to be aware of and set up the hooks in their local repositories. That's why a Git Hooks package is available in almost every package manager for every programming language.
We can use the Git Hooks package to achieve this. However, sometimes many packages are pre-configured and have their own rules or ways of doing things (of course, we can customize that). Besides that, it could be overkill if we only need to run hooks to fix code styling on every commit and have all the pre-configured toolsets that we actually don't really need. It just feels like a waste of resources, right?
So, here I will show you how to set up Git Hooks in our Laravel or many other PHP projects.
Before going further, this step is optional; you can skip it. Click to expand
If you are using VSCode as the editor, the .git folder is invisible by default. So, if you want to show the .git folder, you need to update your VSCode settings. Here is how:
We can set it from the settings.json like this code below:
{
... Your other configuration
"files.exclude": {
"**/.git": false
},
...
}
Or, we can go to the VSCode Settings (UI)
Go to File -> Preferences -> Settings | And remove the **/.git from the list |
---|---|
![]() | ![]() |
First, we can copy the binary files from the .git/hooks folder and paste them into our root folder. Now, we have a hooks folder in our root project folder. It should look like this:
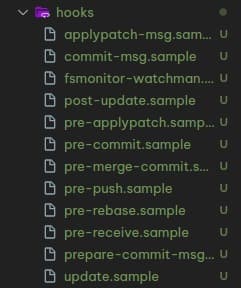
After that, we can do some cleanup to those files, remove that .sample
extension. For example, I only use the pre-commit
hooks. And we can start modifying it. In my case, I use the pre-commit
hooks that will call Laravel Pint to fix some code styling.
#!/bin/sh
staged_files=$(git diff --cached --name-only)
if [ -n "$staged_files" ]; then
./vendor/bin/pint
git add $staged_files
fi
exit 0
By default, our git is still looking for the hooks from the original hooks path .git/hooks
. Now, we need to change the current hooks path to our ./hooks
folder.
To do that, we can run this command to change the hooks path.
git config core.hooksPath hooks
Now, git will read the hooks path from the ./hooks
folder. But, it only happens on our machine. To make sure it can be shared and used seamlessly, we need to put this command somewhere. And, as far as I know, the right place to put this command is in the composer.json
file in the post-autoload-dump scripts section.
{
...
"scripts": {
"post-autoload-dump": [
"Illuminate\\Foundation\\ComposerScripts::postAutoloadDump",
"@php artisan package:discover --ansi",
"git config core.hooksPath hooks"
],
"post-update-cmd": [
"@php artisan vendor:publish --tag=laravel-assets --ansi --force"
],
"post-root-package-install": [
"@php -r \"file_exists('.env') || copy('.env.example', '.env');\""
],
"post-create-project-cmd": [
"@php artisan key:generate --ansi"
]
},
...
}
By doing that, we don't have to worry about the git hooks path. It will "automatically" change to the ./hooks
folder. Our teammate would have the same hooks as we do.
We can actually do more than this example; this fix code style is just to demonstrate that git hooks integration is that easy.
So, I don't have to worry about my other teammates having a different code style; Laravel Pint along with my hooks would handle that.